Guide to Angular BehaviourSubject, ReplaySubject & Subject
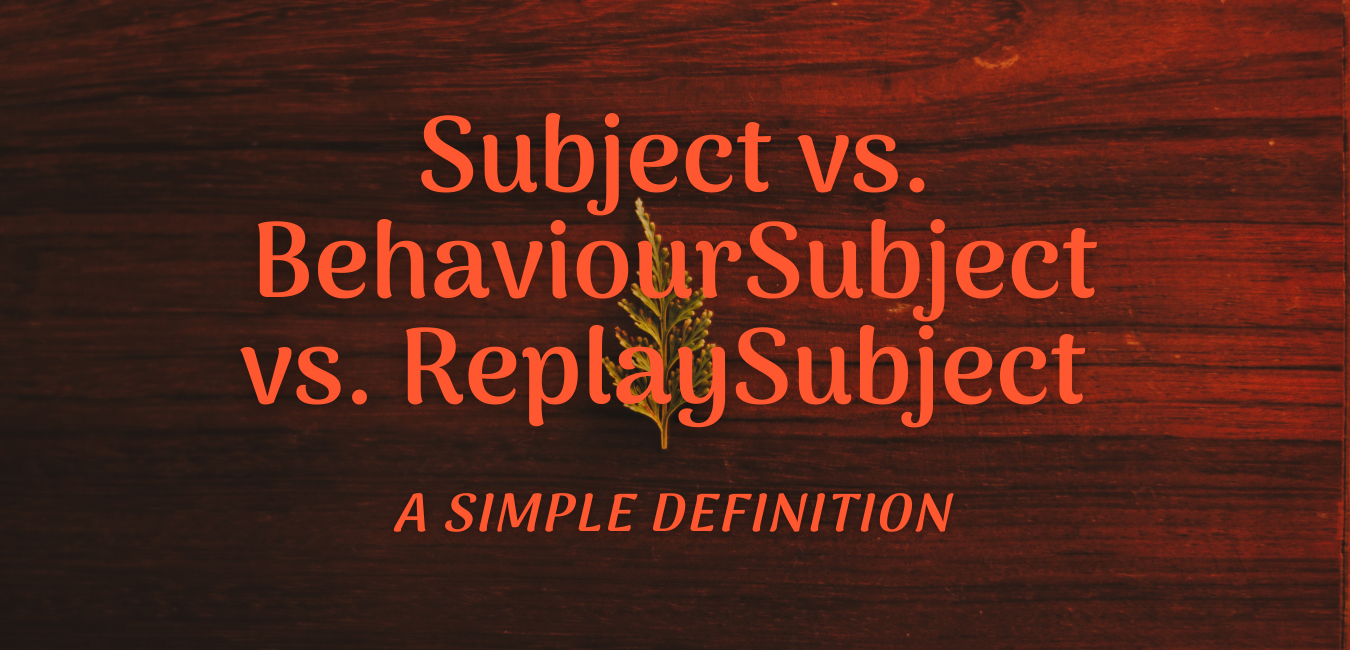
In Angular, reactive programming is a key concept that allows for real-time data updates and management. Subject, BehaviourSubject, and ReplaySubject are all important types of subjects in Angular, each with its own unique use cases and benefits.
In this guide, we'll take a closer look at each of these subject types, comparing and contrasting their features and discussing when it's best to use each.
Whether you're a seasoned Angular developer or just starting out, understanding the differences between these subject types is essential for maximizing the power of Angular's reactive programming.
What is a Subject in Angular?
Subjects are a special kind of observables that enable us to send data to multiple components or services.
The most significant difference between the Subject and the Observable is the casting properties.
A simple observable is a unicast, i.e., it can be cast to a single observer only. It has the power to multicast many observers, making a subject an observer and an observable stream simultaneously until complete notification is emitted. It is noted that subjects do not possess any initial value, and they behave like event emitters.
The code below explains the working of Subject behavior in Angular:
import { Subject } from 'rxjs';
const subject = new Subject<String>();
subject.subscribe({
next: (v) => console.log(`observer 1: ${v}`)
});
subject.subscribe({
next: (v) => console.log(`observer 2: ${v}`)
});
subject.next(“Hello”);
subject.next(“Hi”);
subject.complete();
// Output:
// observer 1: Hello
// observer 2: Hello
// observer 1: Hi
// observer 2: Hi
What is a BehaviourSubject in Angular?
A behavior subject is a type of subject that can be initialized with a value, and it will print the initial value even if it has not received a next().
Moreover, Behavior Subjects keep track of the last values, and whenever there is a new subscriber, it will get the previous value, and log it.
This can be seen in the code below.
import { BehaviorSubject } from 'rxjs';
const subject = new BehaviorSubject(0); // 0 is the initial value
subject.subscribe({
next: (v) => console.log(`observer 1: ${v}`)
});
subject.next(1);
subject.next(2);
subject.subscribe({
next: (v) => console.log(`observer 2: ${v}`)
});
// Output:
// observer 1: 0
// observer 1: 1
// observer 1: 2
// observer 2: 2
The Behavior Subject has a 0 value at the start, and when observer 1 subscribes to it, it will log it. We can see observer 2 subscribe after the value 2, but it still logs it.
This shows the tracking of the last value by Behavior Subject.
What is a ReplaySubject in Angular?
It works similarly to Behavior Subject except that it can keep track of multiple values and reply to them for the new subscribers. Furthermore, you can also specify the window for tracking the older values.
Let us see Reply Subject’s working.
import { ReplaySubject } from 'rxjs';
const subject = new ReplaySubject(3); // stores 3 values for new subscribers
subject.subscribe({
next: (v) => console.log(`observer 1: ${v}`)
});
subject.next(1);
subject.next(2);
subject.next(3);
subject.next(4);
subject.subscribe({
next: (v) => console.log(`observer 2: ${v}`)
});
subject.next(5);
// Output:
// observer 1: 1
// observer 1: 2
// observer 1: 3
// observer 1: 4
// observer 2: 2
// observer 2: 3
// observer 2: 4
// observer 1: 5
// observer 2: 5
As you can see, observer 2 gets 3 previous values after subscribing, as set during Replay Subject's initialization.
When to use Subject in Angular?
Subjects are best used when it is necessary to multicast values to multiple subscribers, but when there is no need to retain any values for new subscribers.
For example, if you have a form that is used to submit data to a server, a Subject can be used to broadcast the form data to multiple components that are interested in the data.
When to use BehaviourSubject in Angular?
BehaviourSubjects are best used when you need to retain the current value for new subscribers.
This is useful when working with data that is updated frequently and needs to be broadcasted to multiple components.
For example, if you have a user profile that is updated frequently, a BehaviourSubject can be used to broadcast the updated profile data to multiple components.
When to use ReplaySubject in Angular?
ReplaySubjects are best used when you need to retain a buffer of values for new subscribers.
This is useful when working with data that is updated frequently and you want new subscribers to receive the most recent values.
For example, if you have stock market data that updates frequently, a ReplaySubject can be used to broadcast the most recent stock market data to new subscribers.
We have seen the Subject, Behavior Subject, and Reply Subject in this article, each having its use case.
- Subjects - are mainly used for multicasting the data.
- Behavior Subjects - get an initializing value and keep track of the last value.
- Reply Subjects - can maintain a buffer of values and pass them to new subscribers.
In conclusion, Subject, BehaviourSubject, and ReplaySubject are essential types of subjects in Angular that play important roles in reactive programming.
Each subject type has its own unique features and use cases, and understanding the differences between them is crucial for making informed decisions about which subject type to use in different situations.